This project uses an Arduino and an ADXL345 accelerometer for Free Fall Detection. When a large acceleration shift characteristic of a free fall is detected, a warning system is activated, which includes an OLED display with the words “Free Fall Detected!” and a buzzer. This system can find applications in safety monitoring and fall detection scenarios.
How it works?
This project uses an Arduino, an ADXL345 accelerometer, and an OLED display to build a Free Fall Detection system. The accelerometer continually measures acceleration in three dimensions. A free fall event takes place when the acceleration surpasses a predetermined threshold. In response, the OLED display displays the message “Free Fall Detected!” and a buzzer sounds a brief alert. The device is customizable by modifying the threshold to suit different applications, making it excellent for safety monitoring and fall detection scenarios. It provides a simple yet effective approach for detecting probable fall accidents in real-time.
Components Required
- ESP32
- ADXL345 Accelerometer Sensor
- 0.96 inch OLED Display
- Buzzer
Introduction to ADXL345 Accelerometer Sensor
The ADXL345 is a flexible 3-axis accelerometer sensor that measures acceleration in three dimensions precisely. It has high resolution (up to 13-bit), a wide range of sensitivity options, and low power consumption, making it perfect for motion, tilt, and vibration detection in a variety of applications. This sensor can communicate through I2C or SPI so it is suitable for use in microcontroller-based projects.
Pinout of ADXL345
This is a pinout diagram of ADXL345 Sensor
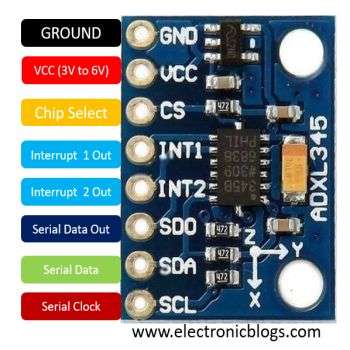
Pin Name | Pin Description |
GND | Ground Pin |
VCC | Power Supply (3V to 6V) |
CS | Chip Select Pin |
INT1 | Interrupt 1 Output Pin |
INT2 | Interrupt 2 Output Pin |
SDO | Serial Data Output Pin |
SDA | Serial Data Input & Output |
SDL | Serial Communication Clock |
Circuit Diagram
This is a simple circuit diagram of Free Fall Detection Using Arduino.
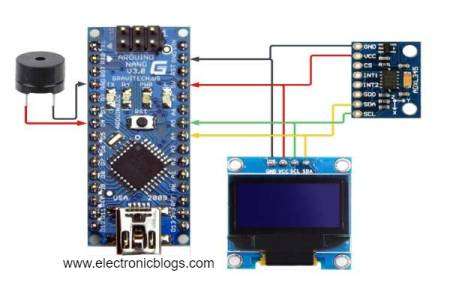
OLED Pin | Arduino Nano Pin |
VCC | 5V Pin |
GND | GND of Nano |
SDA | A4 |
SCL | A5 |
ADXL Pin | ESP32 Pin |
VCC | 5V Pin |
GND | GND of Nano |
SDA | A4 |
SCL | A5 |
ADXL Pin | ESP32 Pin |
VCC | D4 |
GND | GND of Nano |
Physical Connections
This is a physical connection image of Free Fall Detection Using Arduino Nano.
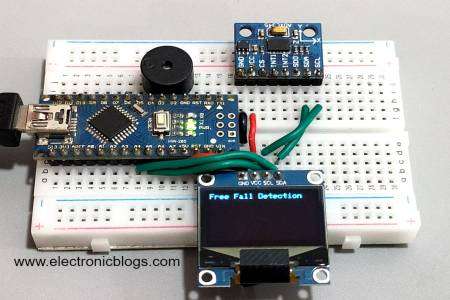
Program/Code:
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <Adafruit_Sensor.h>
#include <Adafruit_ADXL345_U.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
Adafruit_ADXL345_Unified accel = Adafruit_ADXL345_Unified(12345);
const int buzzerPin = 4; // Pin for the buzzer
const float rollThreshold = 20.0; // Adjust this threshold based on your accelerometer readings
void setup() {
Serial.begin(9600);
display.begin(SSD1306_SWITCHCAPVCC, 0x3C); // Initialize the OLED display
display.clearDisplay();
display.setTextColor(WHITE);
display.setTextSize(1);
display.setCursor(0, 0);
display.println("Free Fall Detection");
display.display();
if (!accel.begin()) {
display.clearDisplay();
display.setCursor(10, 20);
display.println("ADXL345 not found!");
display.display();
while (1);
}
accel.setRange(ADXL345_RANGE_16_G);
pinMode(buzzerPin, OUTPUT); // Initialize the buzzer pin
}
void loop() {
sensors_event_t event;
accel.getEvent(&event);
float magnitude = sqrt(pow(event.acceleration.x, 2) + pow(event.acceleration.y, 2) + pow(event.acceleration.z, 2));
if (magnitude >= rollThreshold) {
// Display the free fall message on the OLED display
display.clearDisplay();
display.setTextSize(2);
display.setCursor(0, 0);
display.println("Free Fall Detected!");
display.display();
// Turn on the buzzer
digitalWrite(buzzerPin, HIGH);
// Wait for a short period
delay(1000);
// Turn off the buzzer
digitalWrite(buzzerPin, LOW);
}
// Print the accelerometer values to the serial monitor
Serial.print("Magnitude: ");
Serial.println(magnitude);
// Delay for a short period
delay(50); // Adjust the delay as needed
}
Program Explanation:
Library and Display Setup:
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <Adafruit_Sensor.h>
#include <Adafruit_ADXL345_U.h>
These lines include the libraries required for I2C connection, graphics rendering, and interacting with the ADXL345 accelerometer.
OLED Display and Accelerometer Initialization:
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
Adafruit_ADXL345_Unified accel = Adafruit_ADXL345_Unified(12345);
This part defines the screen dimensions and initialises an Adafruit SSD1306 object for the OLED display. It also generates an Adafruit ADXL345 object for the accelerometer.
Constants and Variables:
const int buzzerPin = 4; // Pin for the buzzer
const float rollThreshold = 20.0; // Adjust this threshold based on your accelerometer readings
These lines define a constant buzzerPin (pin 4) for the buzzer and a rollThreshold value used to assess if a free fall is detected. You can change this threshold based on your accelerometer measurements.
Setup Function:
void setup() {
Serial.begin(9600);
display.begin(SSD1306_SWITCHCAPVCC, 0x3C); // Initialize the OLED display
// ... OLED display setup code ...
if (!accel.begin()) {
// Display an error message if the accelerometer is not found
// ...
while (1);
}
accel.setRange(ADXL345_RANGE_16_G);
pinMode(buzzerPin, OUTPUT); // Initialize the buzzer pin
}
The setup function initializes the serial connection, configures the OLED display, and verifies that the accelerometer (ADXL345) is properly connected. If the accelerometer is not found, an error message appears on the OLED screen and the program enters an indefinite loop. The sensitivity range of the accelerometer is set to 16g, and the buzzer pin is set up as an output.
Main Loop:
void loop() {
sensors_event_t event;
accel.getEvent(&event);
float magnitude = sqrt(pow(event.acceleration.x, 2) + pow(event.acceleration.y, 2) + pow(event.acceleration.z, 2));
if (magnitude >= rollThreshold) {
// Display "Free Fall Detected!" and activate the buzzer
// ...
}
// Print accelerometer values to the serial monitor
// ...
delay(50); // Delay for a short period
}
The loop function reads acceleration data from the ADXL345 accelerometer continuously. The magnitude of the acceleration vector is calculated and compared with the rollThreshold. If the magnitude surpasses the threshold, an alarm appears on the OLED display and the buzzer sounds immediately. The accelerometer data is also printed to the serial monitor for monitoring reasons, and iterations are separated by a short delay.
Conclusion
This project demonstrates a Free Fall Detection system that uses an Arduino and an ADXL345 accelerometer. The applications range from assuring elderly care safety to improving industrial safety, and it can even be used in wearable devices for fall detection. It provides a versatile solution for real-time fall detection and safety enhancement due to the flexibility to modify sensitivity.